java-gui
简介
java-gui不流行的原因:界面不美观、需要jre环境
swing、AWT
AWT
abstract windows
java.awt
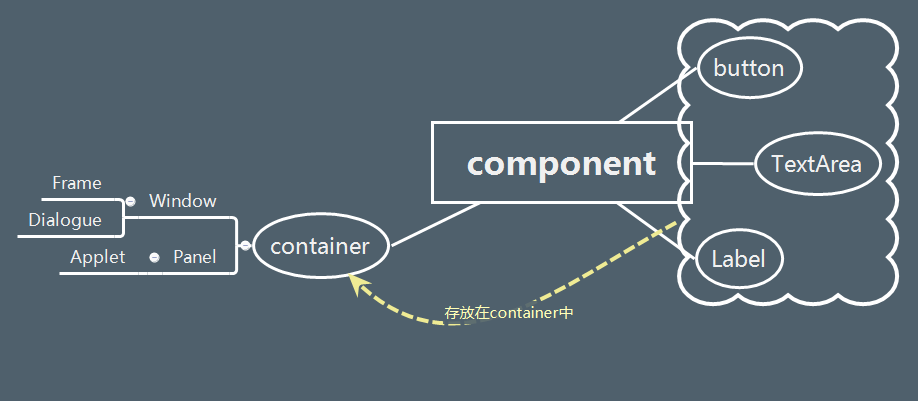
Frame
setBounds设置Location和长宽
setBackground设置Frame的背景颜色
setVisible设置可见性
Frame中可以添加panel
关闭窗口:addWindowListener
1 2 3 4 5 6 7 8
| frame.addWindowListener(new WindowAapter()){ @Override public void windowClosing(WindowEvent e){ System.exit(0); } }
|
panel
三种布局管理器
流式布局
FlowLayout()
一排一排的摆放,第一行摆完了再摆第二行
边界布局
BorderLayout()
中间会比较大,其他的地方比较小
表格布局
GridLayout()
frame.pack()自动填充大小
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42
| import java.awt.*;
public class test { public static void main(String[] args) { Frame frame = new Frame(); frame.setLayout(new GridLayout(2, 1));
frame.setVisible(true); frame.setBounds(200, 200, 400, 400); frame.setBackground(Color.BLACK);
Panel p1 = new Panel(new BorderLayout()); Panel p2 = new Panel(new GridLayout(2, 2)); Panel p3 = new Panel(new BorderLayout()); Panel p4 = new Panel(new GridLayout(2, 1)); p1.add(new Button("WEST-1"), BorderLayout.WEST); p1.add(new Button("EAST-1"), BorderLayout.EAST);
for(int i=0; i<4;i++){ p2.add(new Button("CENTER-"+(i+1))); }
p1.add(p2, BorderLayout.CENTER);
p4.add(new Button("CENTER-5")); p4.add(new Button("CENTER-6"));
p3.add(new Button("WEST-2"), BorderLayout.WEST); p3.add(new Button("EAST-2"), BorderLayout.EAST); p3.add(p4, BorderLayout.CENTER);
frame.add(p1); frame.add(p3); } }
|
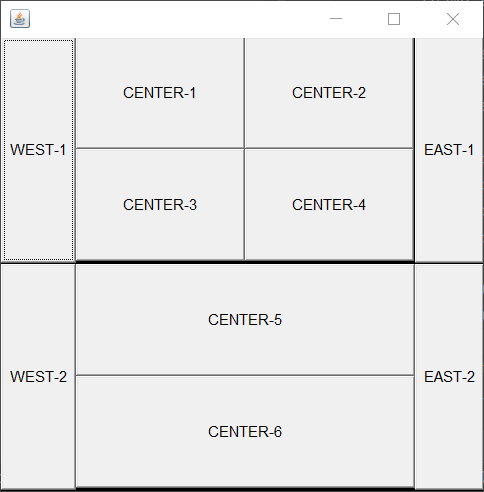
事件监听
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43
| import java.awt.*; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; import java.awt.event.WindowAdapter; import java.awt.event.WindowEvent;
public class testactionlisterner { public static void main(String[] args){ Frame frame = new Frame(); Button button = new Button("test");
MyActionListener myActionListener = new MyActionListener(); button.addActionListener(myActionListener);
frame.add(button, BorderLayout.CENTER); frame.pack(); windowClose(frame);
frame.setVisible(true); }
private static void windowClose(Frame frame){ frame.addWindowListener(new WindowAdapter() { @Override public void windowClosing(WindowEvent e) { super.windowClosing(e); System.exit(0); } }); }
}
class MyActionListener implements ActionListener{
@Override public void actionPerformed(ActionEvent e){ System.out.println("abc"); } }
|
输入框
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35
| import java.awt.*; import java.awt.event.ActionEvent; import java.awt.event.ActionListener;
public class Testtext1 { public static void main(String[] args) { new MyFrame(); } }
class MyFrame extends Frame{ public MyFrame(){ TextField textField = new TextField(); add(textField); textField.setEchoChar('*');
MyActionListener2 myActionListener2 = new MyActionListener2(); textField.addActionListener(myActionListener2);
pack(); setVisible(true); } }
class MyActionListener2 implements ActionListener{ @Override public void actionPerformed(ActionEvent e){
TextField content = (TextField)e.getSource(); System.out.println(content.getText()); } }
|
简易计算器
oop原则:优先使用组合,组合大于继承(继承会增加耦合性 )
1 2 3 4 5 6 7 8 9
| class A extends B{ }
class A{ public B b; }
|
初始代码
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83
| import java.awt.*; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; import java.awt.event.WindowAdapter; import java.awt.event.WindowEvent;
public class TestCalc { public static void main(String[] args) { new Calculator(); } }
class Calculator extends Frame{ public Calculator(){ TextField num1 = new TextField(10); TextField num2 = new TextField(10); TextField num3 = new TextField(20);
Button button = new Button("=");
Label label = new Label("+");
setLayout(new FlowLayout());
add(num1); add(label); add(num2); add(button); add(num3);
MyCalculatorListener myCalculatorListener = new MyCalculatorListener(num1, num2, num3); button.addActionListener(myCalculatorListener);
pack(); setVisible(true); }
private static void windowClose(Frame frame){ frame.addWindowListener(new WindowAdapter() { @Override public void windowClosing(WindowEvent e) { super.windowClosing(e); System.exit(0); } }); } }
class MyCalculatorListener implements ActionListener { private TextField num1, num2, num3;
public MyCalculatorListener(TextField num1, TextField num2, TextField num3){ this.num1 = num1; this.num2 = num2; this.num3 = num3; }
@Override public void actionPerformed(ActionEvent e){ int n1 = Integer.parseInt(num1.getText()); int n2 = Integer.parseInt(num2.getText());
num3.setText(""+(n1+n2));
num1.setText(""); num2.setText("");
} }
|
改为面向对象的写法
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71
| import java.awt.*; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; import java.awt.event.WindowAdapter; import java.awt.event.WindowEvent;
public class TestCalc { public static void main(String[] args) { new Calculator().loadFrame(); } }
class Calculator extends Frame{ TextField num1, num2, num3;
public void loadFrame(){ num1 = new TextField(10); num2 = new TextField(10); num3 = new TextField(20);
Button button = new Button("="); button.addActionListener(new MyCalculatorListener(this));
Label label = new Label("+");
setLayout(new FlowLayout());
add(num1); add(label); add(num2); add(button); add(num3); pack(); setVisible(true); windowClose(this);
}
private static void windowClose(Frame frame){ frame.addWindowListener(new WindowAdapter() { @Override public void windowClosing(WindowEvent e) { super.windowClosing(e); System.exit(0); } }); } }
class MyCalculatorListener implements ActionListener { Calculator calculator = null;
public MyCalculatorListener(Calculator calculator){ this.calculator = calculator; }
@Override public void actionPerformed(ActionEvent e){
int n1 = Integer.parseInt(calculator.num1.getText()); int n2 = Integer.parseInt(calculator.num2.getText()); calculator.num3.setText(""+(n1+n2)); calculator.num1.setText(""); calculator.num2.setText(""); } }
|
使用内部类进行优化:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65
| import java.awt.*; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; import java.awt.event.WindowAdapter; import java.awt.event.WindowEvent;
public class TestCalc { public static void main(String[] args) { new Calculator().loadFrame(); } }
class Calculator extends Frame{ private TextField num1, num2, num3;
public void loadFrame(){ num1 = new TextField(10); num2 = new TextField(10); num3 = new TextField(20);
Button button = new Button("="); button.addActionListener(new MyCalculatorListener());
Label label = new Label("+");
setLayout(new FlowLayout());
add(num1); add(label); add(num2); add(button); add(num3); pack(); setVisible(true); windowClose(this);
}
private static void windowClose(Frame frame){ frame.addWindowListener(new WindowAdapter() { @Override public void windowClosing(WindowEvent e) { super.windowClosing(e); System.exit(0); } }); }
private class MyCalculatorListener implements ActionListener { @Override public void actionPerformed(ActionEvent e){
int n1 = Integer.parseInt(num1.getText()); int n2 = Integer.parseInt(num2.getText()); num3.setText(""+(n1+n2)); num1.setText(""); num2.setText(""); } } }
|
画笔
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25
| import java.awt.*;
public class TestPaint { public static void main(String[] args) { new MyPaint().loadFrame(); } }
class MyPaint extends Frame{
public void loadFrame(){ setBounds(200,200,600,500); setVisible(true); }
@Override public void paint(Graphics g){ g.setColor(Color.BLUE); g.drawOval(100, 100, 100, 100); g.fillOval(100,100,100,100);
} }
|
鼠标监听
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61
| import java.awt.*; import java.awt.event.MouseAdapter; import java.awt.event.MouseEvent; import java.util.ArrayList; import java.util.Iterator;
public class TestMouseListener { public static void main(String[] args) { new MyFrame2("Drawing"); } }
class MyFrame2 extends Frame{
ArrayList points;
public MyFrame2(String title){ super(title); setBounds(200, 200, 400, 300);
points = new ArrayList<>();
this.addMouseListener(new MyMouseListener()); setVisible(true); }
@Override public void paint(Graphics g){ Iterator iterator = points.iterator(); while(iterator.hasNext()){ Point point = (Point) iterator.next(); g.setColor(Color.RED); g.fillOval(point.x, point.y, 10, 10); }
}
public void addPaint(Point point){ points.add(point); }
private static class MyMouseListener extends MouseAdapter{ @Override public void mousePressed(MouseEvent e){ MyFrame2 frame = (MyFrame2) e.getSource(); frame.addPaint(new Point(e.getX(),e.getY()));
frame.repaint();
} } }
|
窗口监听
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31
| import java.awt.*; import java.awt.event.WindowAdapter; import java.awt.event.WindowEvent;
public class TestWindowListener { public static void main(String[] args) { new WindowFrame(); } }
class WindowFrame extends Frame { public WindowFrame(){ setVisible(true); setBounds(200,200,200,200); addWindowListener( new WindowAdapter() { @Override public void windowClosing(WindowEvent e) { System.out.println("closed"); System.exit(0); }
@Override public void windowActivated(WindowEvent e) { System.out.println("activated"); } } ); } }
|
键盘监听
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27
| import java.awt.*; import java.awt.event.KeyAdapter; import java.awt.event.KeyEvent;
public class Testkeylistener { public static void main(String[] args) { new Mykeylistener(); } }
class Mykeylistener extends Frame { public Mykeylistener(){ setVisible(true); setBounds(10,10,100,100); addKeyListener( new KeyAdapter() { @Override public void keyPressed(KeyEvent e) { int keycode = e.getKeyCode(); if(keycode == KeyEvent.VK_UP){ System.out.println("up up up"); } } } ); } }
|
Swing
窗口、面板
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28
| import javax.swing.*; import java.awt.*;
public class JFrameDemo1 { public static void main(String[] args) { new MyJrame1().init(); } }
class MyJrame1 extends JFrame{ public void init(){ setBounds(10,10,100,100); setVisible(true);
JLabel label = new JLabel("hello world"); label.setHorizontalAlignment(SwingConstants.CENTER); add(label);
Container container = getContentPane(); container.setBackground(Color.RED);
setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE); } }
|
弹窗
新建一个弹窗类,然后用事件监听去绑定这个弹窗,在点击按钮之后new我们新写的弹窗类
标签
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46
| import javax.swing.*; import java.awt.*;
public class TestIcon extends JFrame implements Icon{ private int width; private int height;
public TestIcon(){}
public TestIcon(int width, int height){ this.width = width; this.height = height; }
public void init(){ TestIcon icondemo = new TestIcon(15, 15); JLabel icon = new JLabel("icon", icondemo, SwingConstants.CENTER);
Container container = getContentPane(); container.add(icon);
this.setVisible(true); this.setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE); }
public static void main(String[] args) { new TestIcon().init(); }
@Override public void paintIcon(Component c, Graphics g, int x, int y) { g.fillOval(x, y, width, height); }
@Override public int getIconWidth() { return this.width; }
@Override public int getIconHeight() { return this.height; } }
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28
| import javax.swing.*; import java.awt.*; import java.net.URL;
public class ImageIconDemo extends JFrame { public ImageIconDemo(){ JLabel label = new JLabel("ImageIcon"); URL url = ImageIconDemo.class.getResource("timg.jpg");
ImageIcon imageIcon = new ImageIcon(url);
label.setIcon(imageIcon); label.setHorizontalAlignment(SwingConstants.CENTER);
Container container = getContentPane(); container.add(label);
this.setVisible(true); this.setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE); this.setBounds(100,100,200,200); }
public static void main(String[] args) { new ImageIconDemo(); } }
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21
| import javax.swing.*; import java.awt.*;
public class JScollDemo extends JFrame { public JScollDemo() { Container container = getContentPane();
JTextArea textarea = new JTextArea(20,50); textarea.setText("jscoll"); JScrollPane scollpane = new JScrollPane(textarea); container.add(scollpane);
this.setVisible(true); this.setBounds(100,100,300,300); this.setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE); }
public static void main(String[] args) { new JScollDemo(); } }
|
图片按钮、单选框、多选框
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25
| import javax.swing.*; import java.awt.*; import java.net.URL;
public class IconButtonDemo extends JFrame{ public IconButtonDemo() { URL url = IconButtonDemo.class.getResource("timg.jpg"); Icon icon = new ImageIcon(url);
JButton button = new JButton(); button.setIcon(icon); button.setToolTipText("image_button");
Container container = getContentPane(); container.add(button); this.setVisible(true); this.setBounds(100,100,100,100);
}
public static void main(String[] args) { new IconButtonDemo(); } }
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33
| import javax.swing.*; import java.awt.*;
public class JRadioButtonDemo extends JFrame { public JRadioButtonDemo(){ Container container = this.getContentPane();
JRadioButton rb1 = new JRadioButton("rb1"); JRadioButton rb2 = new JRadioButton("rb2"); JRadioButton rb3 = new JRadioButton("rb3");
ButtonGroup buttonGroup = new ButtonGroup(); buttonGroup.add(rb1); buttonGroup.add(rb2); buttonGroup.add(rb3);
container.add(rb1, BorderLayout.NORTH); container.add(rb2, BorderLayout.CENTER); container.add(rb3, BorderLayout.SOUTH);
this.setVisible(true); this.setBounds(100,100,100,100); this.setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE); }
public static void main(String[] args) { new JRadioButtonDemo(); } }
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22
| import javax.swing.*; import java.awt.*;
public class JCheckBoxDemo extends JFrame { public JCheckBoxDemo() throws HeadlessException { Container container = getContentPane();
JCheckBox check1 = new JCheckBox("check1"); JCheckBox check2 = new JCheckBox("check2");
container.add(check1, BorderLayout.NORTH); container.add(check2, BorderLayout.SOUTH);
this.setVisible(true); this.setBounds(100,100,100,100); this.setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE); }
public static void main(String[] args) { new JCheckBoxDemo(); } }
|
下拉框、列表框
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24
| import javax.swing.*; import java.awt.*;
public class ComboboxDemo extends JFrame { public ComboboxDemo() throws HeadlessException { Container container = getContentPane();
JComboBox box = new JComboBox(); box.addItem(null); box.addItem("1"); box.addItem("2"); box.addItem("3");
container.add(box);
this.setVisible(true); this.setSize(200,200); this.setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE); }
public static void main(String[] args) { new ComboboxDemo(); } }
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31
| import javax.swing.*; import java.awt.*; import java.util.Vector;
public class JListDemo extends JFrame { public JListDemo() throws HeadlessException { Container container = getContentPane();
Vector contents = new Vector(); JList jList = new JList(contents);
container.add(jList);
contents.add("1"); contents.add("2"); contents.add("3");
this.setVisible(true); this.setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE); this.setSize(100,100); }
public static void main(String[] args) { new JListDemo(); } }
|
文本框、密码框、文本域
TextArea文本域可以换行,TextField文本框不能换行
1 2
| JPasswordField pass = new JPasswordField(); pass.setEchoChar("*");
|
SnakeGame
https://github.com/risuxx/SnakeGame